所谓的代理者是指一个类别可以作为其它东西的接口。代理者可以作任何东西的接口:网络连接、存储器中的大对象、文件或其它昂贵或无法复制的资源。著名的代理模式例子为引用计数(英语:reference counting)指针对象。
代理模式
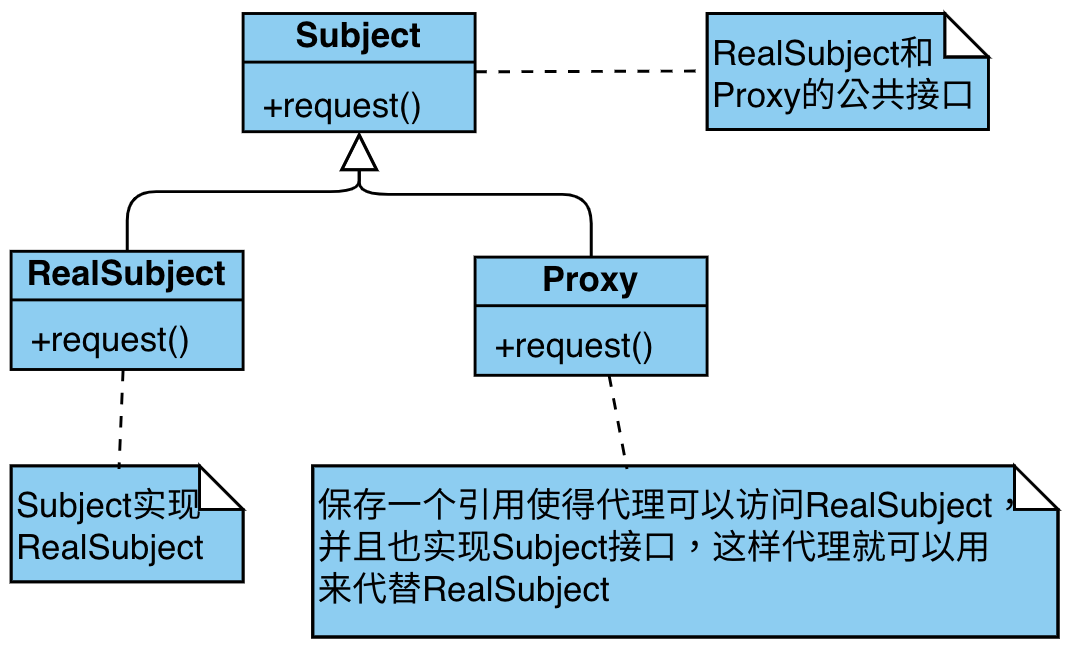
为别人做嫁衣
UML类图
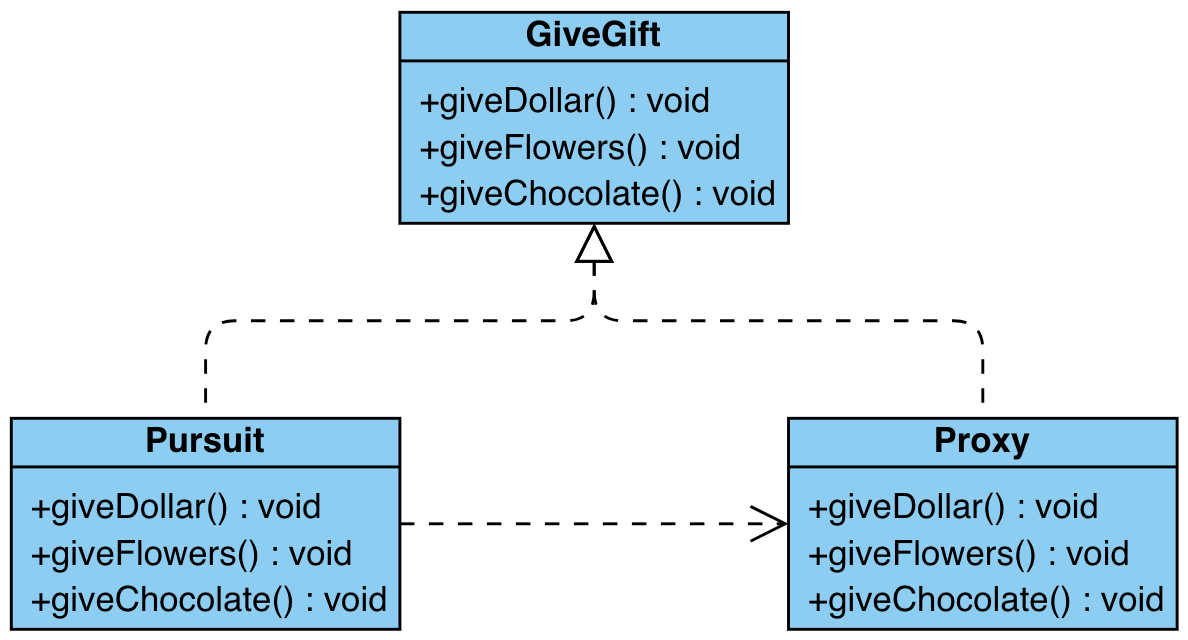
代码实现
GiveGift
1 2 3 4 5 6 7
| package com.lei.designpatterns.proxy;
public interface GiveGift { void giveDollar(); void giveFlowers(); void giveChocolate(); }
|
SchoolGirl
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.lei.designpatterns.proxy;
public class SchoolGirl { private String name;
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public SchoolGirl(String name){ this.name = name; } }
|
Pursuit
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.lei.designpatterns.proxy;
public class Pursuit implements GiveGift { private SchoolGirl mm;
public Pursuit(SchoolGirl mm){ this.mm = mm; }
@Override public void giveDollar() { System.out.println("Give dollar."); }
@Override public void giveFlowers() { System.out.println("Give flowers."); }
@Override public void giveChocolate() { System.out.println("Give chocolate."); } }
|
Proxy
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.lei.designpatterns.proxy;
public class Proxy implements GiveGift { private Pursuit gg;
public Proxy(SchoolGirl mm){ gg = new Pursuit(mm); }
@Override public void giveDollar() { this.gg.giveDollar(); }
@Override public void giveFlowers() { this.gg.giveFlowers(); }
@Override public void giveChocolate() { this.gg.giveChocolate(); } }
|