普通的工厂方法模式通常伴随着对象的具体类型与工厂具体类型的一一对应,客户端代码根据需要选择合适的具体类型工厂使用。然而,这种选择可能包含复杂的逻辑。这时,可以创建一个单一的工厂类,用以包含这种选择逻辑,根据参数的不同选择实现不同的具体对象。
计算器
实现一个具有加、减、乘、除的计算器。
UML类图
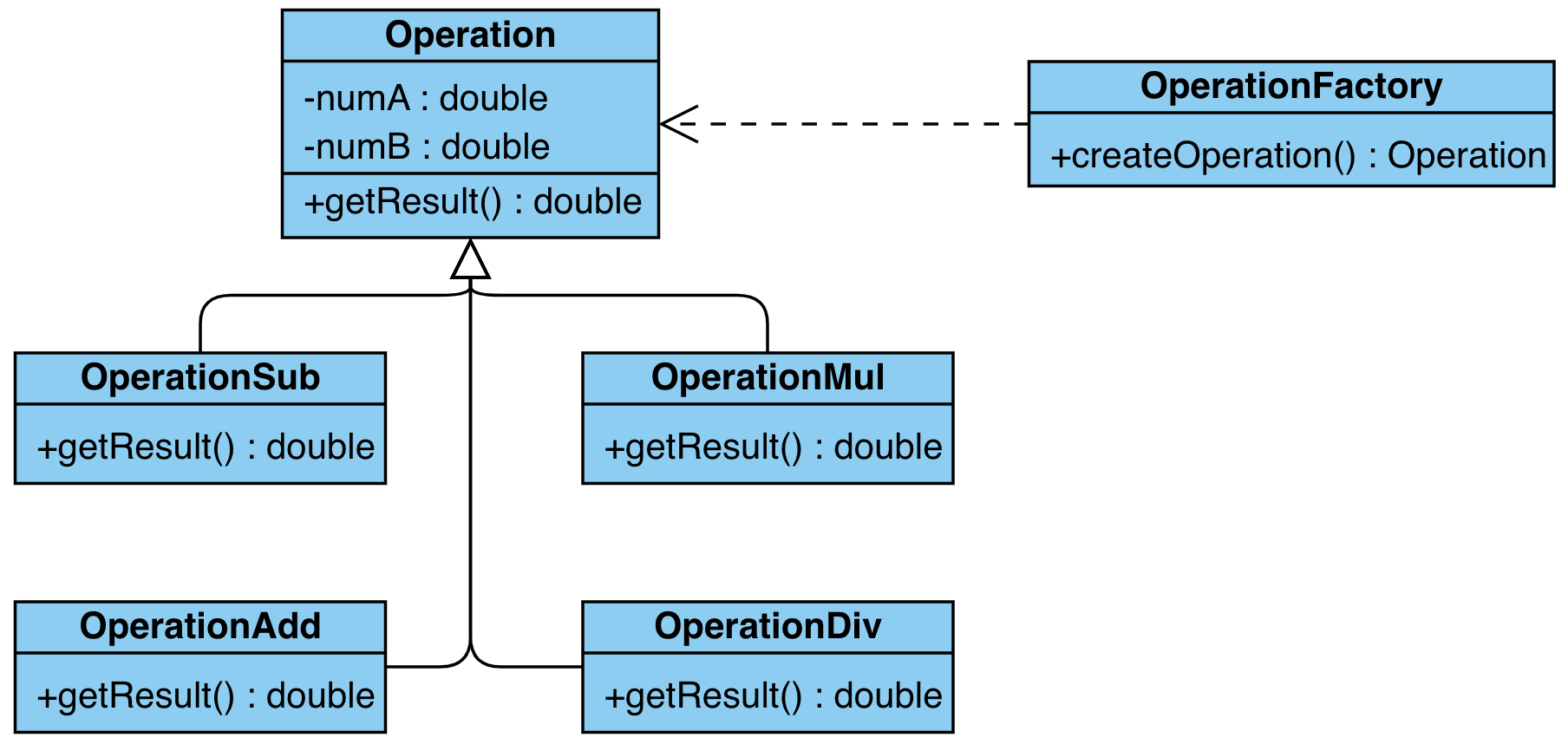
代码样例
Operation类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| package com.lei.designpatterns.simplefactory;
public abstract class Operation { protected double numA;
protected double numB;
public double getNumA() { return numA; }
public void setNumA(double numA) { this.numA = numA; }
public double getNumB() { return numB; }
public void setNumB(double numB) { this.numB = numB; }
public abstract double getResult(); }
|
OperationAdd类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.simplefactory;
public class OperationAdd extends Operation{ @Override public double getResult() { return this.numA + this.numB; } }
|
OperationSub类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.simplefactory;
public class OperationSub extends Operation { @Override public double getResult() { return this.numA - this.numB; } }
|
OperationMul类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.simplefactory;
public class OperationMul extends Operation { @Override public double getResult() { return this.numA * this.numB; } }
|
OperationDiv类
1 2 3 4 5 6 7 8 9 10 11
| package com.lei.designpatterns.simplefactory;
public class OperationDiv extends Operation { @Override public double getResult() { if (this.numB < 0.000001){ throw new ArithmeticException("Divide by zero."); } return this.numA / this.numB; } }
|
OperationFactory类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.lei.designpatterns.simplefactory;
import com.lei.payment.java.base.algorithm.exception.AlgorithmException;
public class OperationFactory { public static Operation createOperation(String operate){ if (operate == null){ throw new NullPointerException("Operate is empty."); } switch (operate){ case "+": return new OperationAdd(); case "-": return new OperationSub(); case "*": return new OperationMul(); case "/": return new OperationDiv(); default: throw new AlgorithmException("Not support operate."); } } }
|