策略模式是指对象有某个行为,但是在不同的场景中,该行为有不同的实现算法。
策略模式
UML类图
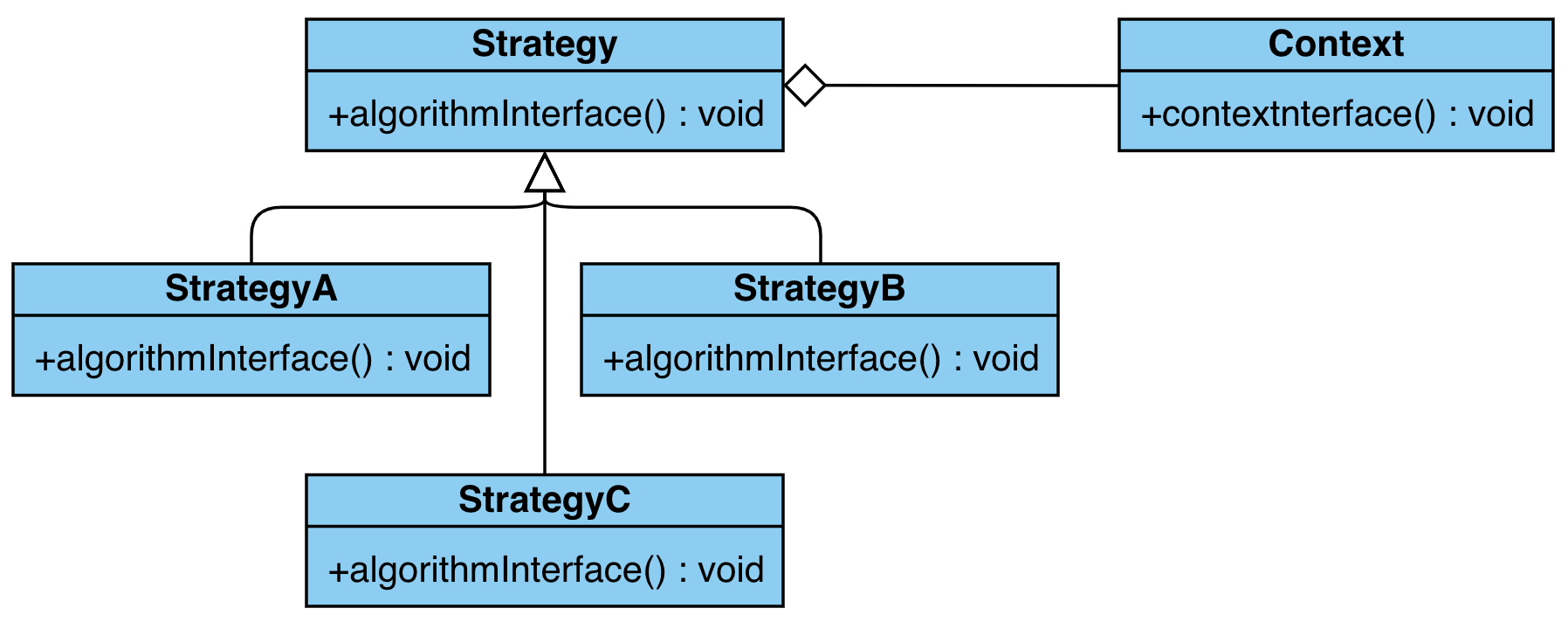
代码实现
Strategy类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.strategy;
public abstract class Strategy {
public abstract void algorithmInterface(); }
|
StrategyA类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.strategy;
public class ConcreteStrategyA extends Strategy{ @Override public void algorithmInterface() { System.out.println("Algorithm a.") ; } }
|
StrategyB类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.strategy;
public class ConcreteStrategyB extends Strategy{ @Override public void algorithmInterface() { System.out.println("Algorithm b.") ; } }
|
StrategyC类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.strategy;
public class ConcreteStrategyC extends Strategy{ @Override public void algorithmInterface() { System.out.println("Algorithm c.") ; } }
|
Context类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| package com.lei.designpatterns.strategy;
public class Context {
private Strategy strategy;
public Context(Strategy strategy){ this.strategy = strategy; }
public void contextInterface(){ this.strategy.algorithmInterface(); } }
|
商场促销
营业员在收银台,需要根据所购买商品的单价与数量,向客户收费。并且需要考虑在不同的场景下,会有不同的打折情况,比如8折或者满减。
简单工厂实现
UML类图
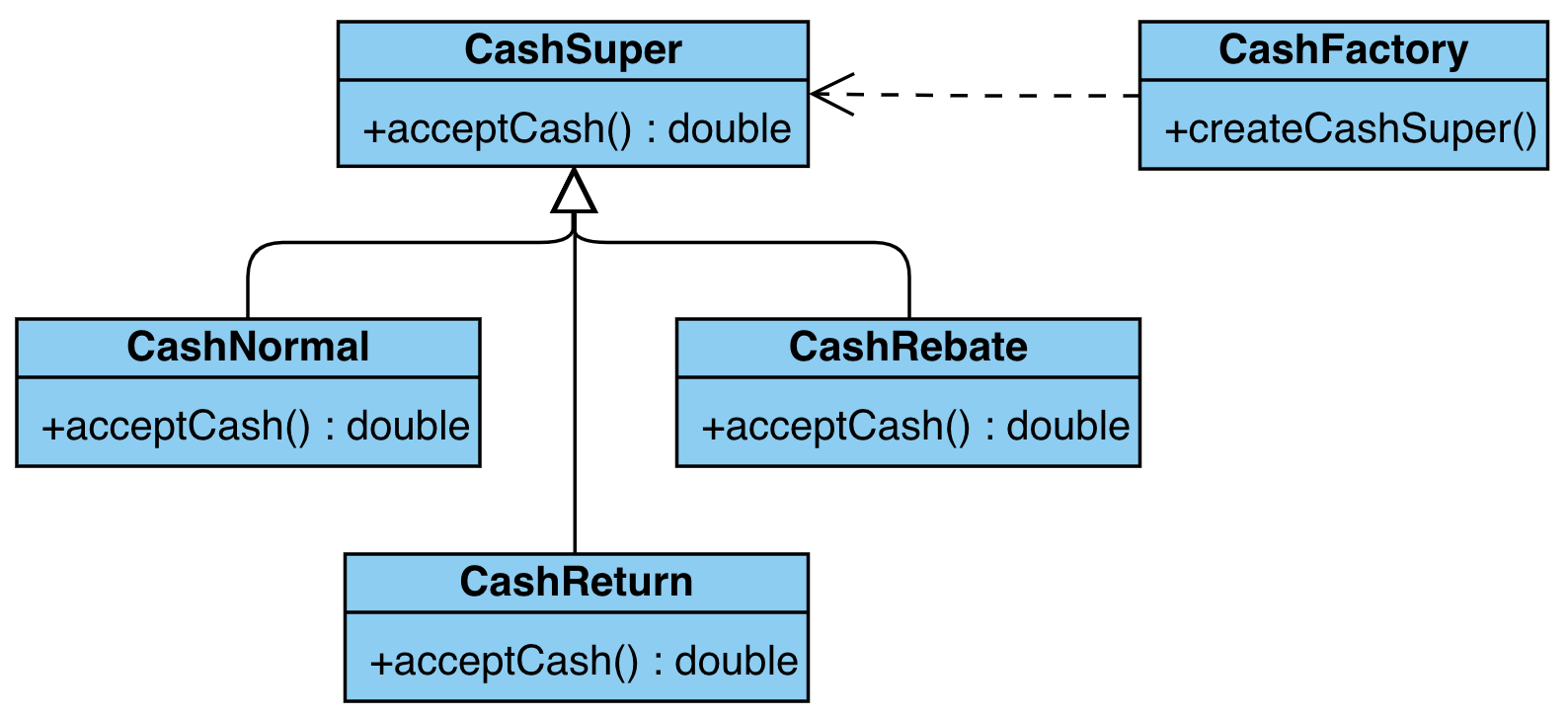
代码实现
CashSuper类
1 2 3 4 5 6 7 8 9 10 11
| package com.lei.designpatterns.strategy;
public abstract class CashSuper {
public abstract double acceptCash(double money); }
|
CashNormal类
1 2 3 4 5 6 7 8
| package com.lei.designpatterns.strategy;
public class CashNormal extends CashSuper{ @Override public double acceptCash(double money) { return money; } }
|
CashRebate类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.lei.designpatterns.strategy;
public class CashRebate extends CashSuper{
private double cashRebate = 1d;
public CashRebate(String moneyRebate){ this.cashRebate = Double.parseDouble(moneyRebate); }
@Override public double acceptCash(double money) { return this.cashRebate * money; } }
|
CashReturn类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.lei.designpatterns.strategy;
public class CashReturn extends CashSuper {
private double moneyCondition = 0.0d;
private double moneyReturn = 0.0d;
public CashReturn(String moneyCondition, String moneyReturn){ this.moneyCondition = Double.parseDouble(moneyCondition); this.moneyReturn = Double.parseDouble(moneyReturn); }
@Override public double acceptCash(double money) { double result = money; if (money > this.moneyCondition){ result = money - Math.floor(money / this.moneyCondition) * this.moneyReturn; } return result; } }
|
CashFactory类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| package com.lei.designpatterns.strategy.simplefactory;
import com.lei.payment.java.base.algorithm.exception.AlgorithmException;
public class CashFactory { public static CashSuper createCashFactory(String type){ CashSuper cs = null; switch (type){ case "Normal": cs = new CashNormal(); break; case "Rebate": cs = new CashRebate("0.8"); break; case "Return": cs = new CashReturn("300","100"); break; default: throw new AlgorithmException("Not support type."); } return cs; } }
|
策略实现
UML类图
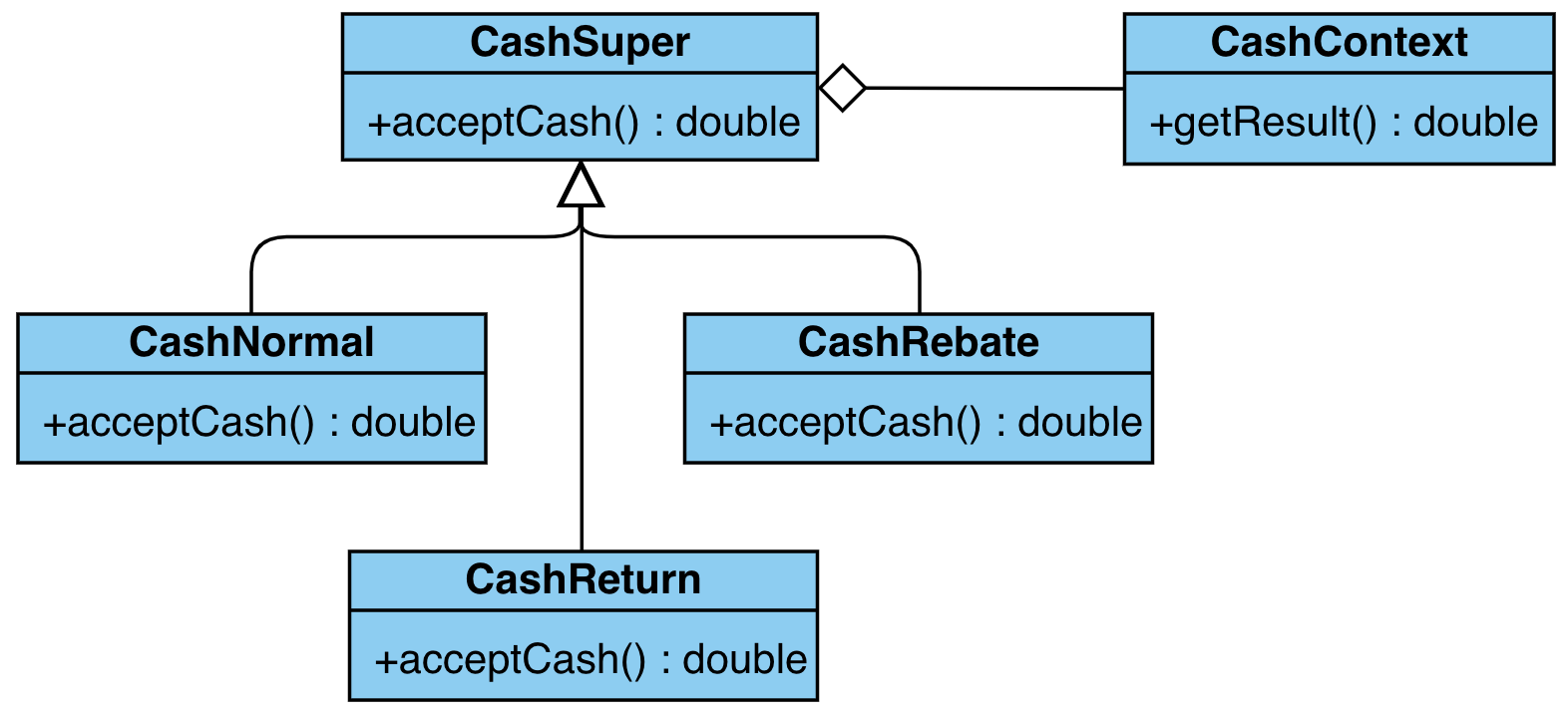
代码实现
CashContext类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package com.lei.designpatterns.strategy.strategy;
import com.lei.designpatterns.strategy.CashSuper;
public class CashContext { private CashSuper cs;
public CashContext(CashSuper cs) { this.cs = cs; }
public double getResult(double money){ return this.cs.acceptCash(money); } }
|
策略与工厂结合
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.lei.designpatterns.strategy;
import com.lei.payment.java.base.algorithm.exception.AlgorithmException;
public class CashContext { private CashSuper cs = null;
public CashContext(String type){ switch (type){ case "Normal": this.cs = new CashNormal(); break; case "Rebate": this.cs = new CashRebate("0.8"); break; case "Return": this.cs = new CashReturn("300","100"); break; default: throw new AlgorithmException("Not support type."); } }
public double getResult(double money){ return this.cs.acceptCash(money); } }
|